Spoken is a free SDK for voice controlled apps. Improve your user's experience with easy to use Human Interface.
Step 1Import Spoken
You can import the spoken module in your app easily using the following example.
npm i spoken
import spoken from './node_modules/spoken/build/spoken.js';
spoken.say('Should I turn the hallway light on?').then( speech => {
spoken.listen().then( transcript =>
console.log("Answer: " + transcript) )
} )
spoken.say('Should I turn the hallway light on?').then( speech => {
spoken.listen().then( transcript =>
console.log("Answer: " + transcript) )
} )
Step 2Text-to-Speech
Synthetic voices are pretty good these days. You can still tell they are robot voices.
Turn text into real-world spoken voice. The voice is synthetic. You can pick from a few different voices too.
// Hello World
spoken.say('Hello World.');
// Say a quick message as Guy Fieri
spoken.say( 'Looks like your on a trip to flavor town.', 'Daniel' );
// Speak with Damayanti's voice
spoken.say( 'I am princess of the Vidarbha Kingdom.', 'Damayanti' );
Step 3Promises and Await
This SDK Supports modern async programming.
// Promise
spoken.say('Hello World.').then( v => console.log('Done talking.') );
// Async/Await
await spoken.say('Hello World.');
console.log('Done talking.');
Step 4Voice Selection
Synthetic voices are selectable using the spoken.voices method. There is a default voice that is included. You may want to pick a different voice. The following examples show you how to select a differnt voice.
Get list of English voices.
// List of English Speaking Voices
spoken.recognition.language = navigator.language || 'en-US';
let voices = (await spoken.voices()).filter( v => !v.lang.indexOf('en') );
// Use the First Voice
spoken.say( 'Welcome to flavor town.', voices[0] );
// Speak with each English voice to sample your favorites
(await spoken.voices())
.filter( voice => voice.lang.indexOf('en') == 0 )
.map( voice => voice.name )
.forEach( voice =>
spoken.say( 'Welcome to flavor town.', voice ).then(
speech => console.log(speech.voice.name)
)
);
Step 5Listening
Writing a document with your voice or chatting with your friend hands-free is possible when you enable continuous listening mode.
First you should check to see if the browser has access to the Speech-to-Text capability.
const available = spoken.listen.available();
if (available) console.log('Hurray voice transcription is available!');
// Async/Await
console.log(await spoken.listen());
// or Promise style
spoken.listen()
.then( transcript => console.log(transcript))
.catch( error => console.warn(error.message))
// We can only have one concurrent listener.
// So you can catch if there is an error.
spoken.listen()
.then( transcript => console.log(transcript))
.catch( error => console.warn(error.message))
spoken.listen.on.partial( ts => console.log(ts) );
spoken.listen()
.then( ts => console.log("Partial: " + ts))
.catch( error => console.warn(error.message))
spoken.listen.on.start( voice => { console.log('Started Listening') } );
spoken.listen.on.end( voice => { console.log('Ended Listening') } );
spoken.listen.on.error( voice => { console.log('Error Listening') } );
spoken.listen.stop();
spoken.listen.on.end(continueCapture);
spoken.listen.on.error(continueCapture);
startCapture();
function startCapture() {
spoken.listen({ continuous : true }).then( transcript =>
console.log("Captured transcript: " + transcript)
).catch( e => true );
}
async function continueCapture() {
await spoken.delay(300);
if (spoken.recognition.continuous) startCapture();
}
function stopCapture() {
spoken.recognition.continuous = false;
spoken.listen.stop();
}
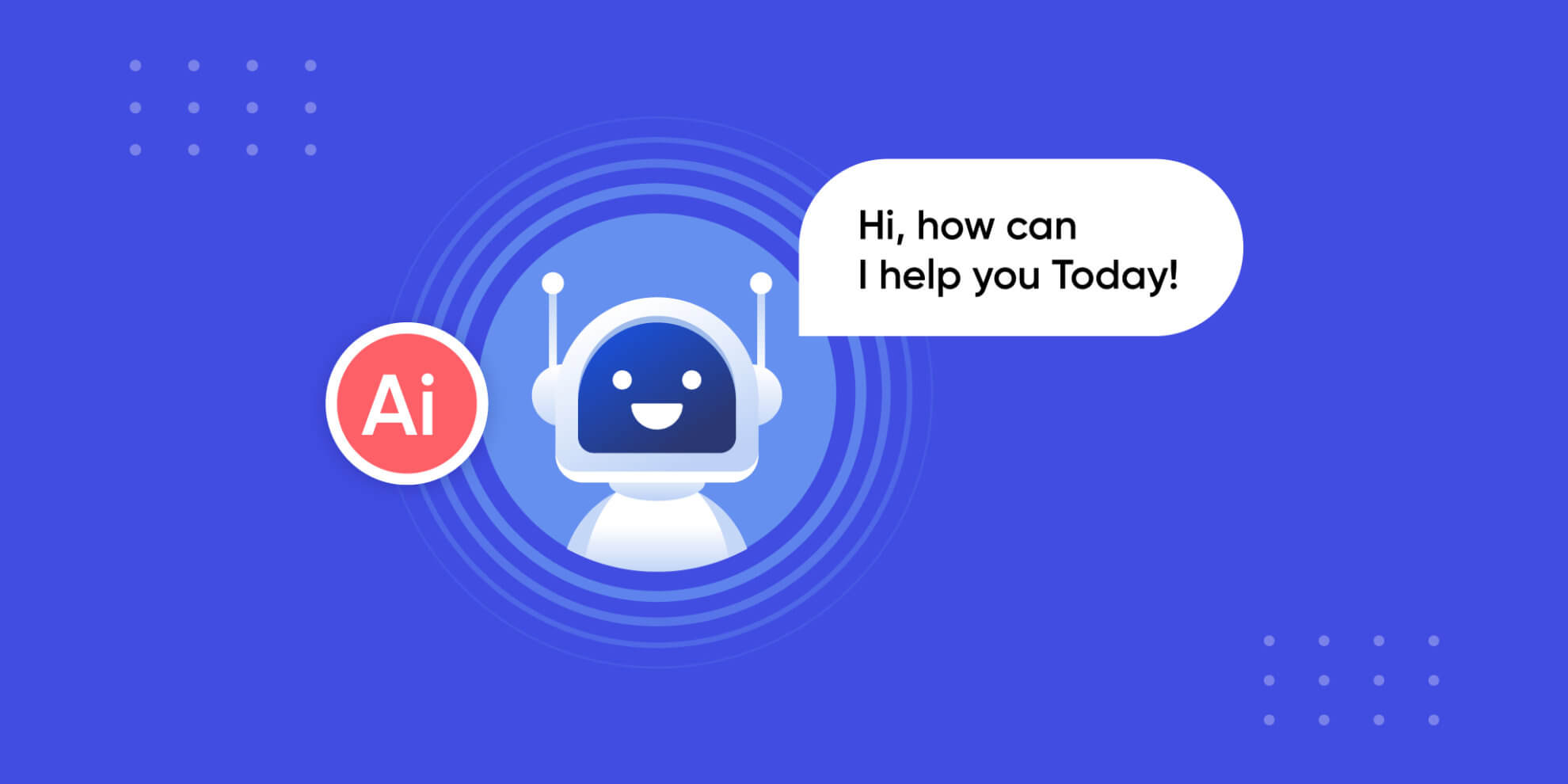
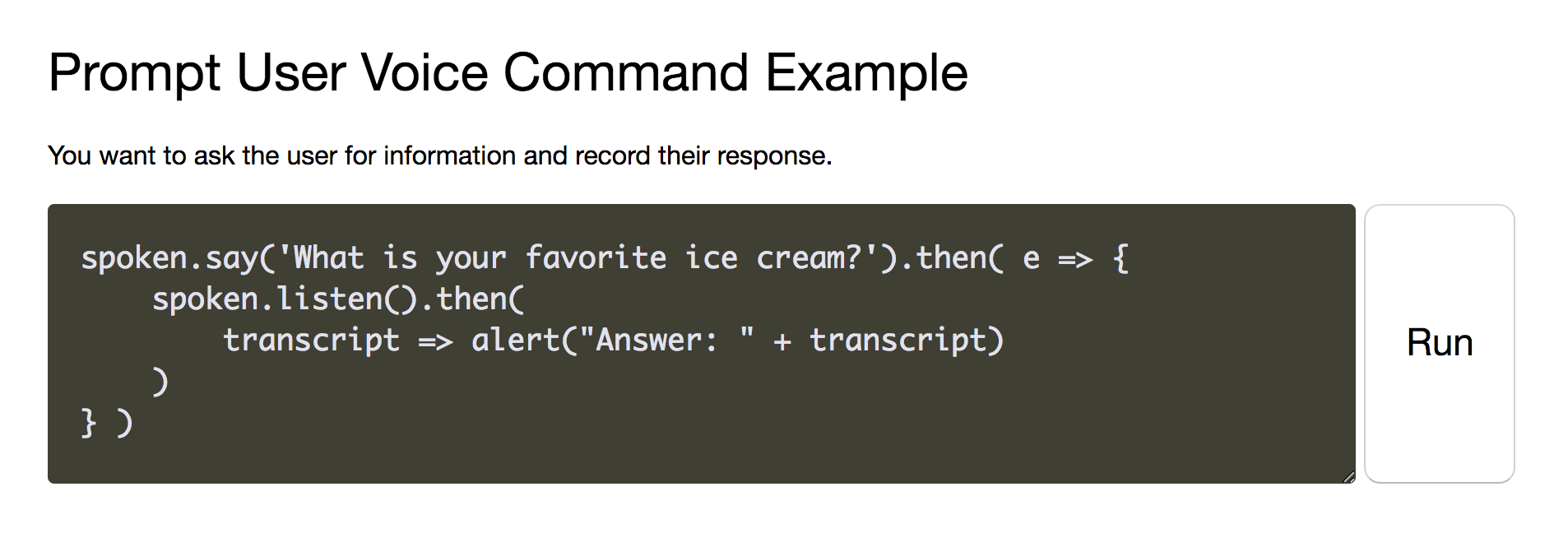
Note: Compatible on Android / iOS / Linux / Windows / MacOS.