You can start making a 1-to-1 call with Direct call or create a room to start a group call with Group call by installing Signal WebRtc SDK for Android.
Step 1Configure ProGuard
When you build your APK with minifyEnabled true, add the following line to the module's ProGuard rules file.
# Call Calls SDK
-keep class com.Call.calls.** { *; }
-keep class org.webrtc.** { *; }
-dontwarn org.webrtc.**
-keepattributes InnerClasses
Step 2Authenticate a user
To make and receive a 1-to-1 call or start a group call, authenticate a user to Call server by using their user ID through the authenticate() method.
// The USER_ID below should be unique to your application.
AuthenticateParams params = new AuthenticateParams(USER_ID)
.setAccessToken(ACCESS_TOKEN);
Call.authenticate(params, new AuthenticateHandler() {
@Override
public void onResult(User user, CallException e) {
if (e == null) {
// The user has been authenticated successfully and is connected to Your server.
...
}
}
});
Step 3Make a call
You are now ready to make your first 1-to-1 call. To make a call, provide the calleeās user ID into the Call.dial() method. To choose initial call configuration such as as audio or video capabilities, video settings, and mute settings, use the CallOptions object.
DialParams params = new DialParams(CALLEE_ID);
params.setVideoCall(true);
params.setCallOptions(new CallOptions());
DirectCall call = CallCall.dial(params, new DialHandler() {
@Override
public void onResult(DirectCall call, CallException e) {
if (e == null) {
// The call has been created successfully.
}
}
});
call.setListener(new DirectCallListener() {
@Override
public void onEstablished(DirectCall call) {
}
@Override
public void onConnected(DirectCall call) {
}
@Override
public void onEnded(DirectCall call) {
}
});
Step 4Receive a call
You can accept or decline an incoming call. To accept an incoming call, use the directCall.accept() method. To decline a call, use the directCall.end() method. If the call is accepted, a media session will automatically be established by the Calls SDK.
Call.addListener(UNIQUE_HANDLER_ID, new CallListener() {
@Override
public void onRinging(DirectCall call) {
call.setListener(new DirectCallListener() {
@Override
public void onEstablished(DirectCall call) {
}
@Override
public void onConnected(DirectCall call) {
}
@Override
public void onEnded(DirectCall call) {
}
@Override
public void onRemoteAudioSettingsChanged(DirectCall call) {
}
});
call.accept(new AcceptParams());
}});
Step 5Device token
You can receive a 1-to-1 call by adding the user's device token to the server. You can add the token by using the Call.registerPushToken() method.
Call.registerPushToken(PUSH_TOKEN, true, new CompletionHandler() {
@Override
public void onResult(@Nullable Exception e) {
if (e == null) {
// The push token is registered successfully.
}
}});
Step 6Encryption
Encrypting a WebRTC call involves implementing encryption and decryption mechanisms to secure the communication between peers. WebRTC does not inherently provide encryption for calls, so you'll need to implement encryption and decryption using existing encryption libraries and methods. Below is a high-level approach to encrypt a WebRTC call in Android using Java.
import javax.crypto.*;
import javax.crypto.spec.SecretKeySpec;
import java.security.SecureRandom;
public class EncryptionUtil {
private static final String AES_ALGORITHM = "AES";
private static final int AES_KEY_SIZE = 128;
public static SecretKey generateAESKey() throws Exception {
SecureRandom secureRandom = new SecureRandom();
byte[] key = new byte[AES_KEY_SIZE / 8];
secureRandom.nextBytes(key);
return new SecretKeySpec(key, AES_ALGORITHM);
}
public static byte[] encryptAES(byte[] data, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance(AES_ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data);
}
public static byte[] decryptAES(byte[] encryptedData, SecretKey secretKey) throws Exception {
Cipher cipher = Cipher.getInstance(AES_ALGORITHM);
cipher.init(Cipher.DECRYPT_MODE, secretKey);
return cipher.doFinal(encryptedData);
}
}
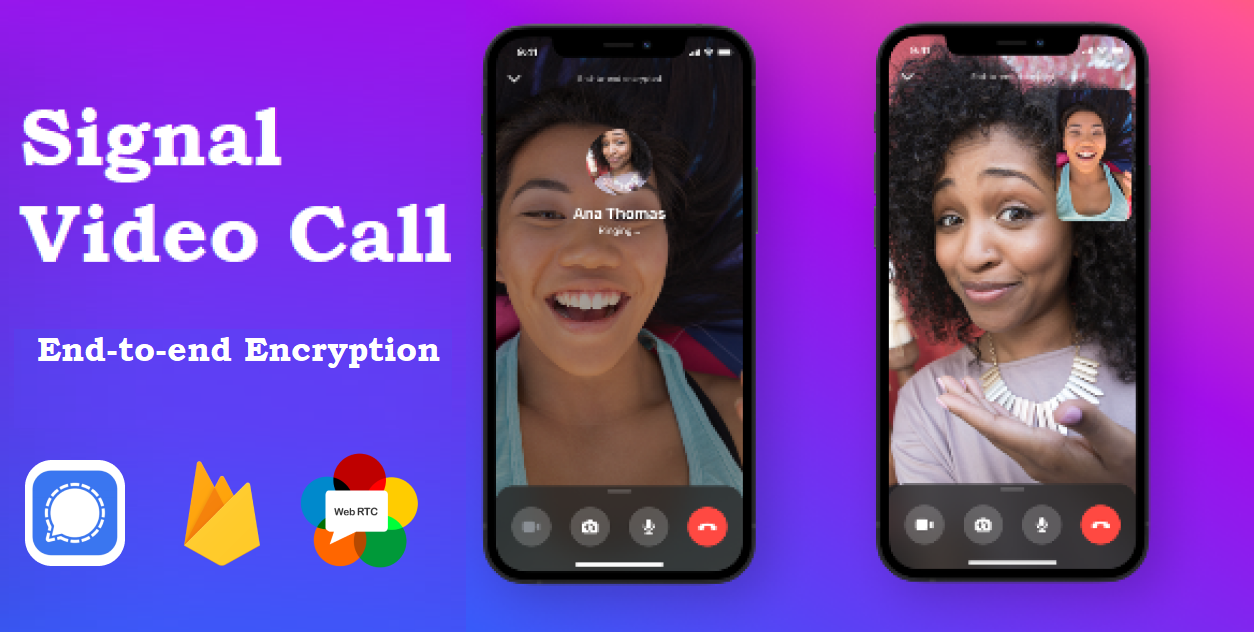
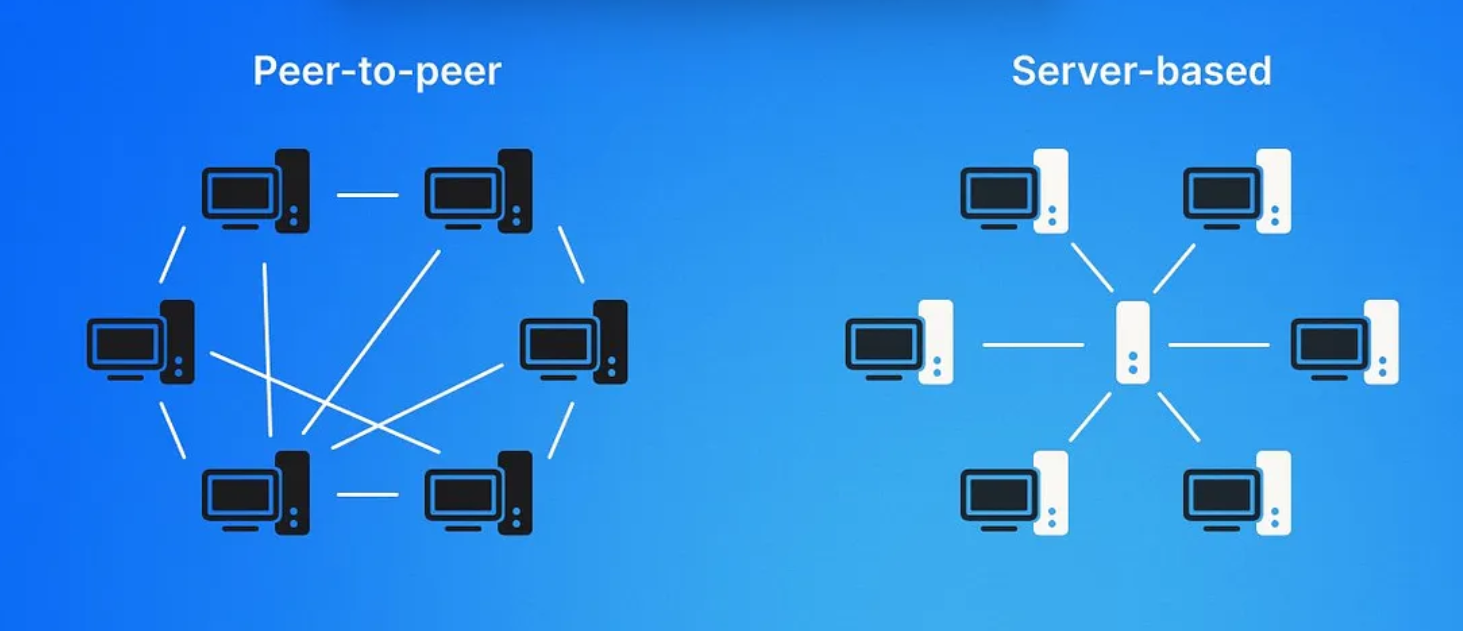
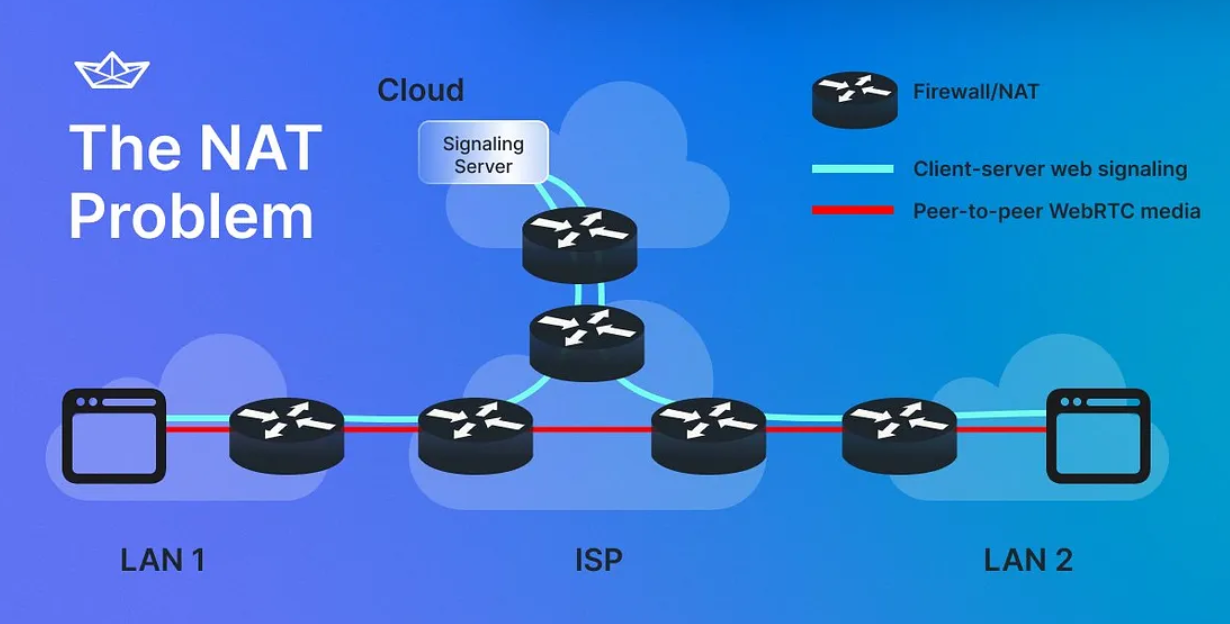
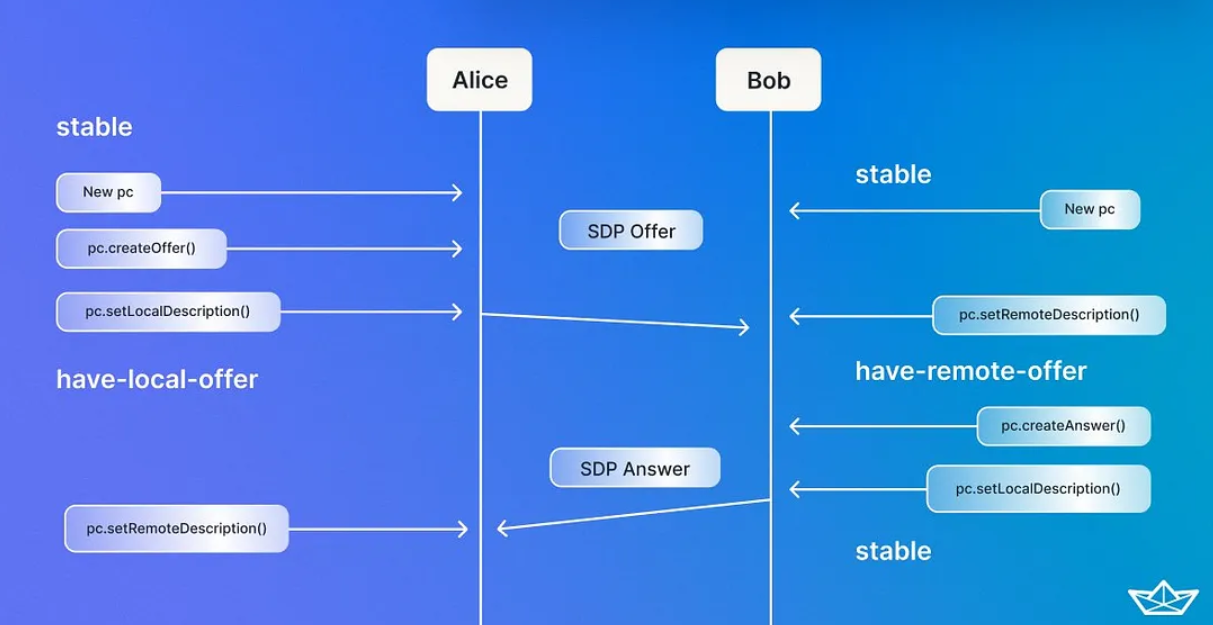
Note: Share the room ID with other users for them to enter the room from the client app.